|
Distance measurement with an ultrasonic sensor can also be carried out in simulation mode. To do this, you must enter the coordinates of the object (target) in the graphics window.
Example 4: Ultrassonic sensor simulation
The robot should travel to the obstacle. If the distance is less than 30 cm, it moves backwards a short distance and then forwards again.
For the simulation, use a horizontal bar as the obstacle, which you create with the following context: |
|
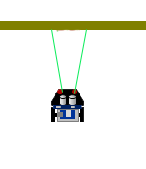 |
target = [[200, 10], [-200, 10], [-200, -10], [200, -10]]
RobotContext.useTarget("sprites/bar0.gif", target, 250, 100)
The first line contains the coordinates of the corner points of the object (a rectangle with the centre at (0,0)). In the graphics window, the object is displayed at the position (250, 100) with the image bar0.gif. The images are included in the TigerJython distribution (Image library).
from mbrobot import *
#from mbrobot_plus import *
target = [[200, 10], [-200, 10], [-200, -10], [200, -10]]
RobotContext.useTarget("sprites/bar0.gif", target, 250, 100)
forward()
repeat:
d = getDistance()
if d < 30:
backward()
delay(2000)
else:
forward()
delay(100)
►Copy to clipboard
In the simulated EV3, the ultrasonic sensor is placed on top of the brick and not at the front. This is why the robot moves a little closer to the beam.
Example 5: Search object in simulation mode
A robot with an ultrasonic sensor rotates slowly around the location. When it detects an object, it moves towards it and stops briefly in front of it.
You write a searchTarget() function that defines the search process: The robot turns to the right in small steps, measuring the distance dist. If it detects an object (dist != -1), it turns a little further and interrupts the search process witht break.
|
|
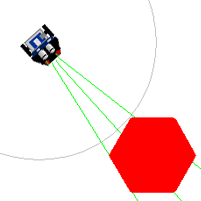 |
Danach fährt er mit Hilfe einer zweiten repeat-Schleife so lange vorwärts, bis die Distanz zum Objekt kleiner als 30 ist. Mit dem Befehl setBeamAreaColor() kannst du den Suchvorgang besser veranschaulichen.
from mbrobot import *
target = [[50,0], [25,43], [-25,43], [-50,0],[-25,-43], [25,-43]]
RobotContext.useTarget("sprites/redtarget.gif", target, 400, 400)
def searchTarget():
repeat:
right()
delay(50)
dist = getDistance()
if dist != -1:
right()
delay(600)
break
setBeamAreaColor(Color.green)
setSpeed(10)
searchTarget()
forward()
repeat:
dist = getDistance()
print(dist)
if dist < 20:
stop()
break
►Copy to clipboard
It then moves forwards with the help of a second repeat loop until the distance to the object is less than 30. You can use the setBeamAreaColor() function to better illustrate the search process..
|